Perievent#
The perievent module allows to re-center time series and timestamps data around a particular event as well as computing events (spikes) trigger average.
Show code cell content
import pynapple as nap
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
custom_params = {"axes.spines.right": False, "axes.spines.top": False}
sns.set_theme(style="ticks", palette="colorblind", font_scale=1.5, rc=custom_params)
Peri-Event Time Histogram (PETH)#
stim = nap.Tsd(
t=np.sort(np.random.uniform(0, 1000, 50)),
d=np.random.rand(50), time_units="s"
)
ts1 = nap.Ts(t=np.sort(np.random.uniform(0, 1000, 2000)), time_units="s")
The function compute_perievent
align timestamps to a particular set of timestamps.
peth = nap.compute_perievent(
timestamps=ts1,
tref=stim,
minmax=(-0.1, 0.2),
time_unit="s")
print(peth)
Index rate ref_times
------- -------- -----------
0 3.33333 62.98
1 3.33333 134.39
2 nan 200.43
3 3.33333 234.91
4 13.33333 268.83
5 6.66667 273.7
6 nan 285.74
... ... ...
43 nan 792.33
44 3.33333 808.76
45 nan 848.03
46 nan 937.43
47 nan 968.58
48 6.66667 993.16
49 6.66667 999.76
The returned object is a TsGroup
. The column ref_times
is a
metadata column that indicates the center timestamps.
Raster plot#
It is then easy to create a raster plot around the times of the
stimulation event by calling the to_tsd
function of pynapple to “flatten” the TsGroup peth
.
plt.figure(figsize=(10, 6))
plt.subplot(211)
plt.plot(np.mean(peth.count(0.01), 1) / 0.01, linewidth=3, color="red")
plt.xlim(-0.1, 0.2)
plt.ylabel("Rate (spikes/sec)")
plt.axvline(0.0)
plt.subplot(212)
plt.plot(peth.to_tsd(), "|", markersize=20, color="red", mew=4)
plt.xlabel("Time from stim (s)")
plt.ylabel("Stimulus")
plt.xlim(-0.1, 0.2)
plt.axvline(0.0)
<matplotlib.lines.Line2D at 0x7f5f13cbfa10>
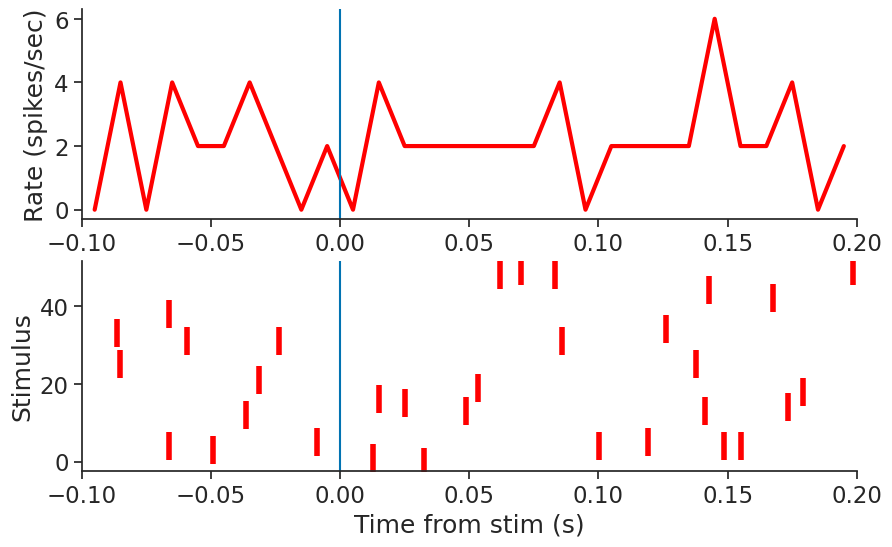
The same function can be applied to a group of neurons.
In this case, it returns a dict of TsGroup
.
Event trigger average#
The function compute_event_trigger_average
compute the average feature around a particular event time.
Show code cell content
group = {
0: nap.Ts(t=np.sort(np.random.uniform(0, 100, 10))),
1: nap.Ts(t=np.sort(np.random.uniform(0, 100, 20))),
2: nap.Ts(t=np.sort(np.random.uniform(0, 100, 30))),
}
tsgroup = nap.TsGroup(group)
eta = nap.compute_event_trigger_average(
group=tsgroup,
feature=stim,
binsize=0.1,
windowsize=(-1, 1))
print(eta)
Time (s) 0 1 2
---------- ------- ------- -------
-1.0 0.06392 0.08522 0.08522
-0.9 0.06392 0.08522 0.08522
-0.8 0.06392 0.08522 0.08522
-0.7 0.06392 0.08522 0.08522
-0.6 0.08522 0.08522 0.08522
-0.5 0.08522 0.08522 0.08522
-0.4 0.08522 0.08522 0.08522
... ... ... ...
0.4 0.08522 0.08522 0.08522
0.5 0.08522 0.08522 0.08522
0.6 0.08522 0.08522 0.08522
0.7 0.08522 0.08522 0.08522
0.8 0.08522 0.08522 0.08522
0.9 0.08522 0.08522 0.08522
1.0 0.08522 0.08522 0.08522
dtype: float64, shape: (21, 3)
Peri-Event continuous time series#
The function nap.compute_perievent_continuous
align a time series of any dimensions around events.
Show code cell content
features = nap.TsdFrame(t=np.arange(0, 100), d=np.random.randn(100,6))
events = nap.Ts(t=np.sort(np.random.uniform(0, 100, 5)))
perievent = nap.compute_perievent_continuous(
timeseries=features,
tref=events,
minmax=(-1, 1))
print(perievent)
Time (s)
---------- -------------------------------
-1 [[ 1.086675 ... -0.684018] ...]
0 [[-2.176375 ... 0.049402] ...]
1 [[-1.494644 ... 1.989631] ...]
dtype: float64, shape: (3, 5, 6)
The object perievent is now of shape (number of bins, (dimensions of input), number of events):
print(perievent.shape)
(3, 5, 6)